What is the meaning of Object-Oriented Programming (OOP)?
Object-oriented programming (OOP) is a modeling system and one of the classifications of programming languages built on the concept of "objects" which can contain data and code. Where "data" denotes fields, attributes, or properties and "code" is referred to as the methods or procedures.
Object-oriented programming is about modeling a system as a collection of objects, which each represent some particular aspect of the system. Objects contain both functions (or methods) and data. An object provides a public interface to other code that wants to use it, but it maintains its private internal state: which means that other parts of the system don't have to care about what is going on inside the object. Object-oriented programming is faster and easier to execute and provides a clear structure for the programs.
OOP helps to keep the C++ code DRY "Don't Repeat Yourself", and makes the code easier to maintain, modify and debug. Programming languages like C++, Java, Python, etc support object-oriented programming to a greater or lesser degree, combined with imperative, procedural programming.
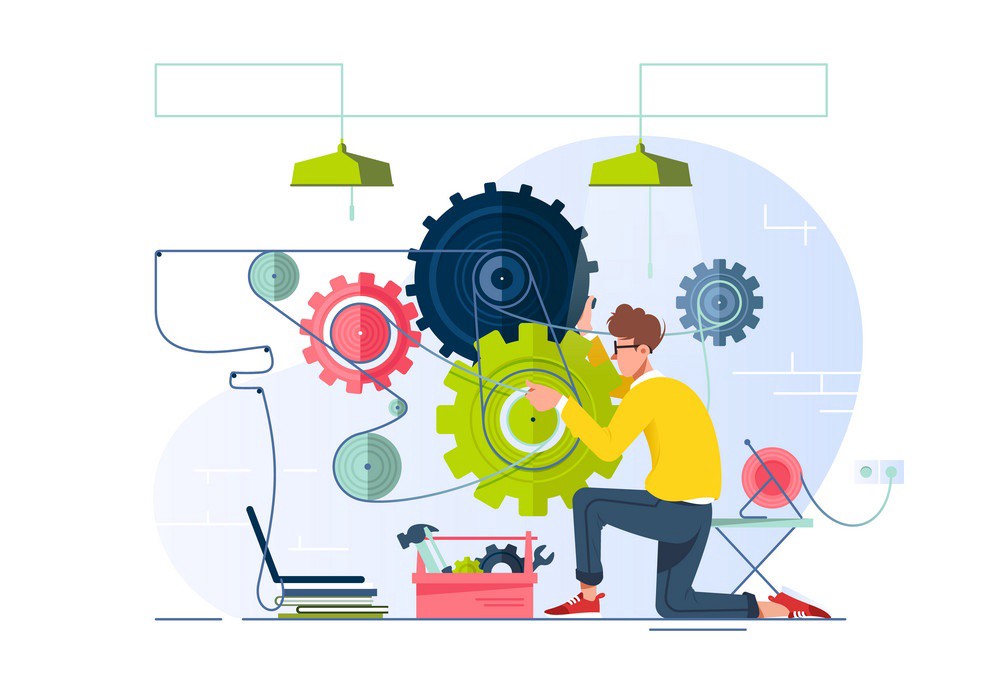
What are the concepts/ principles of Object-Oriented Programming?
There are four main concepts/ principles of Object-oriented programming (OOP), known as Abstraction, Encapsulation, Inheritance, and Polymorphism.
Understand these 2 terms before starting the concepts:
Class: A class is a collection of objects that defines a set of properties that is common to all objects of a particular type. It can also be called a blueprint for creating objects. A class entails class name, modifiers, and body.
Object: An object is defined as an instance of a class and contains real-life entities. For instance, for a class called Animals, Its objects will be a cat, dog, elephant, etc. Each object has its own identity, attribute, and behavior.
1. Abstraction
Is also known as Data Hiding, where we hide a portion of data and show only the relevant part to the final user. Abstraction helps isolate the impact of changes made to the code so that if something goes wrong, the change will only affect the implementation details of a class and not the outside code.
Let's take a real-life example to understand the concept better when we press our finger on a biometric device to open a gate or click on the press the biometric feature on our phone, it automatically opens the system/door. We understand and know the technology on the surface but might not know how it works inside or might not have an urge to understand how it works. Data Abstraction works similarly, as it shows or reflects only the relevant part and hides the less relevant data on purpose.
In C++ data abstraction can be accomplished in 2 different ways-
- using class
- using header file
2. Encapsulation
Encapsulation is the concept of wrapping together data & information in a single unit to save it from manipulation and external excess. The motive behind encapsulation is to hide and bind the data, functions, and logic into a single unit, the class, using access specifiers.
By doing so, we can hide private details of a class from the outside world and only expose functionality that is important for interfacing with it.
Example: (Source: ProCodeGuide )
“An encapsulated class "Employee" in which we have specified properties Name & Total Experience to be specified by User. Employee Id is generated internally in class and generation logic is not exposed to the user also user can only read Employee Id and cannot change it. Similarly, salary is also calculated internally in class, and the user can access employee salary by the GetSalary method”.
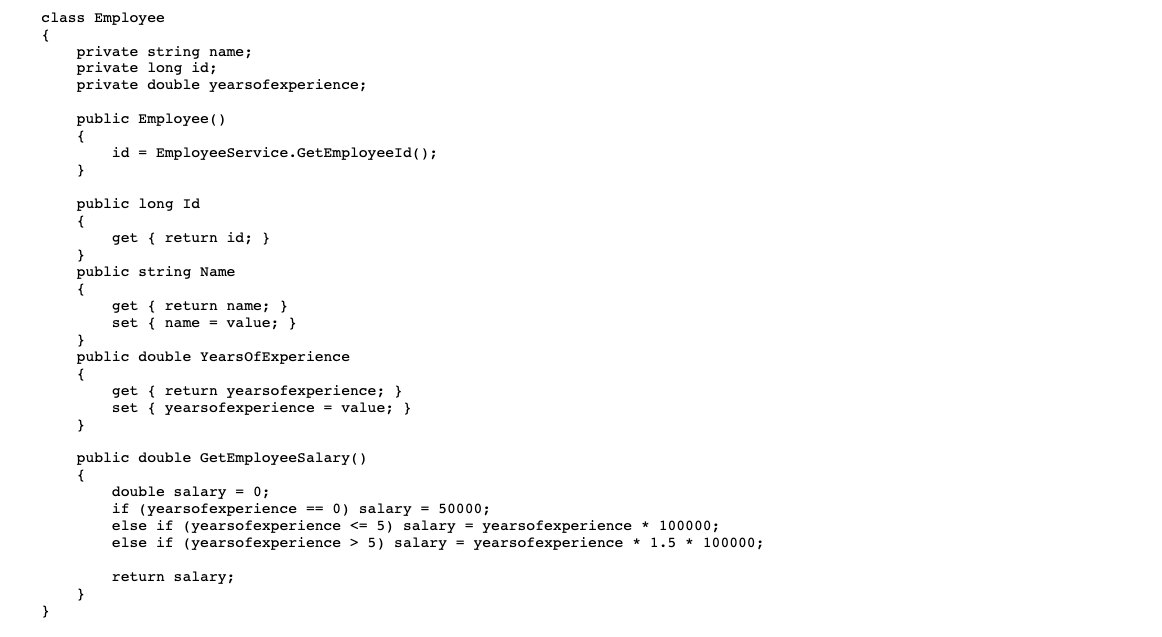
3. Inheritance
Under the Inheritance mechanism, a class inherits the features (fields and methods) of another class. As it's very important in Object-Oriented Programming to allow the reusability factor from the functionality perspective.
- Super Class: The class whose features are inherited is known as superclass (or a base class or a parent class).
- Sub Class: The class that inherits the other class is known as a subclass (or a derived class, extended class, or child class). The subclass can add its fields and methods in addition to the superclass fields and methods.
- Reusability: Using an existing set of codes while creating a new class.
4. Polymorphism
It is considered one of the important features of Object-Oriented Programming. Polymorphism allows us to perform a single action in different ways. In other words, polymorphism allows you to define one interface and have multiple implementations.
What are Object-Oriented Programming design patterns?
A design pattern is a general repeatable solution to a commonly occurring problem in software design. A design pattern isn't a finished design that can be transformed directly into code. It is a description or template for how to solve a problem that can be used in many different situations. Object-Oriented Programming design patterns are divided into 3 categories; Behavioral Patterns, Creational Patterns, and Structural Design Patterns.
Following is the list of all the design patterns used in OOP.
How does Procedural Oriented Programming differ from Object-Oriented Programming?
Following are the important differences between Procedural Oriented Programming (POP) and Object-Oriented Programming (OOP)
What are the examples of Object-Oriented Programming Languages?
- JAVA
- PYTHON
- PHP
- C++
- PERL
- LISP
- COBOL
- C#
What are the advantages of Object-Oriented Programming?

1. Reusability
OOP allows us to use the existing codes through inheritance. We can easily acquire the existing functionality and improve on it without having to rewrite the code again.
2. Modularity
In OOP, it’s easy to modify or troubleshoot the program if a problem occurs or if we need to add a new feature or enhance the existing one.
3. Flexibility
OOP helps us with flexible programming using the polymorphism feature. As polymorphism takes many forms and can work with many objects. It can save us from writing different functions for each object.
4. Maintainability
Maintaining code is easier as it is easy to add new classes, objects, etc without much restructuring or changes.
5. Data and Information Hiding
OOP aids us in data hiding and keeps the information safe from leaking. Only the data that is required for the functioning of the program are exposed to the user by hiding intrinsic or extra detail.